On top of what we have from Part 1 we need:
The prerequisites are:
- Clean install of JBoss Application Server 5.1.0 GA
- JBoss ESB 4.7 GA installed into the clean installation of App Server
- Installed Ant at version 1.7.1 or higher
- Installed Maven at version 2.2.1 or higher
- Installed BPELUnit 1.1.0 Standalone version
Preparation
If you navigate to the directory with Riftsaw source code you will see a sub-directory called “integration-tests” which contains sources of the tests, build scripts for running them and the generated test reports. Take a look in the RIFTSAW_SRC/integration-tests/src/test/resources/samples/ directory where you can find sub-directories for each test, containing BPEL and WSDL files, XML files with messages and the ant build script (build.xml) that compiles the test and makes everything ready for its execution.
To prepare the integration test to use BPELUnit we need to set up a few things:
- Create a “bpelunit” folder in the test sub-directory.
- Create a BPELUnit test suite file in this folder.
- Create a build file that deploys, runs and undeploys the new tests and generates the reports.
- Modify the “deploy” target of the existing build script to compile the BPELUnit tests and deploy them into appropriate directory.
- Add an “antcall” command to integration-tests' pom.xml maven script to call bpelunit build file during the integration-tests phase.
In the first part we were using the quickstart Hello World sample, so we will stick to this one and show how to test it via BPELUnit automatically during the integration tests.
First we create a BPELUnit test suite file "Quickstart_bpel_hello_world.bpts" in RIFTSAW_SRC/integration-tests/src/test/resources/samples/Quickstart_bpel_hello_world/bpelunit/. We will use the very same file we created using Eclipse, so you can just copy it. In case you didn't follow the first part of this article you can find the test suite source code there and just paste it into the new file.
Next we need to create the build script for this new test. Open a new file “bpelunit_build.xml” and paste the code below.
<project name="Quickstart_bpel_hello_world" default="test" basedir=".">
<description>
${ant.project.name}
${line.separator}
</description>
<property name="version" value="1" />
<property name="server.dir" value="${org.jboss.as.home}/server/${org.jboss.as.config}"/>
<property name="conf.dir" value="${server.dir}/conf"/>
<property name="deploy.dir" value="${server.dir}/deploy"/>
<property name="server.lib.dir" value="${server.dir}/lib"/>
<property name="test.dir" value="${basedir}/target/tests/${ant.project.name}" />
<property name="reports.dir" value="${basedir}/target/bpelunit-reports" />
<property name="sample.jar.name" value="${ant.project.name}-${version}.jar" />
<path id="lib.path">
<fileset dir="${bpelunit.home}/lib">
<include name="*.jar" />
</fileset>
</path>
<condition property="reportsDir.unavailable">
<not>
<available file="${reports.dir}" type="dir" />
</not>
</condition>
<target name="deploy">
<echo>Deploy ${ant.project.name}</echo>
<copy file="${test.dir}/${sample.jar.name}" todir="${deploy.dir}" />
<echo>Waiting 5 seconds to ensure proper deployment</echo>
<sleep seconds="5" />
</target>
<target name="undeploy">
<echo>Undeploy ${ant.project.name}</echo>
<delete file="${deploy.dir}/${sample.jar.name}" />
<echo>Waiting 5 seconds to ensure proper undeployment</echo>
<sleep seconds="5" />
</target>
<target name="create.reportsDir" if="reportsDir.unavailable" >
<mkdir dir="${reports.dir}"/>
</target>
<target name="test">
<antcall target="deploy" />
<echo>Running BPEL Unit test for ${ant.project.name}</echo>
<tstamp>
<format property="timestamp" pattern="yyyy-MM-dd_HH:mm:ss" />
</tstamp>
<typedef name="bpelunit" classname="org.bpelunit.framework.ui.ant.BPELUnit">
<classpath refid="lib.path" />
</typedef>
<antcall target="create.reportsDir" />
<bpelunit testsuite="${test.dir}/${ant.project.name}.bpts" bpelunitdir="${bpelunit.home}">
<output style="PLAIN" file="${reports.dir}/${ant.project.name}.${timestamp}.txt" />
<output style="XML" file="${reports.dir}/${ant.project.name}.${timestamp}.xml" />
</bpelunit>
<antcall target="undeploy" />
</target>
</project>
The important target in this script is “test” which first deploys the process into Riftsaw running in JBoss AS, then runs the test and generates reports (in plain-text and XML format) and then undeploys the process.
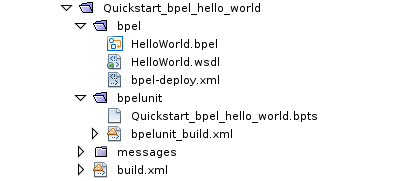
Now we have two files in the Quickstart_bpel_hello_world/bpelunit directory, the test suite and the build script we've just created. We want these files to be copied into the deploy directory for the test to be properly executed. On top of that we need to copy the WSDL file as well. To do this, open the "build.xml" file located in the test's root directory and at the end of the first "target" element add:
<echo>Deploy BPELUnit files for ${ant.project.name}</echo>
<copy todir="${deploy.dir}/${ant.project.name}">
<fileset dir="${test.dir}/bpelunit"/>
</copy>
<copy todir="${deploy.dir}/${ant.project.name}">
<fileset dir="${test.dir}/bpel">
<include name="*.wsdl" />
</fileset>
</copy>
This ensures that the WSDL file, the test suite and the BPELUnit build script will be copied into the deploy directory, which is RIFTSAW_SRC/integration-tests/target/tests/Quickstart_bpel_hello_world/.
One last thing remaining is modifying the "pom.xml" in integration-tests root directory. Open this file and in the project/build/plugins/plugin/executions element create a new "execution" node that will look like this:
<execution>
<id>test-riftsaw-bpelunit</id>
<phase>integration-test</phase>
<configuration>
<tasks>
<ant antfile="${basedir}/target/tests/Quickstart_bpel_hello_world/bpelunit_build.xml" />
</tasks>
</configuration>
<goals>
<goal>run</goal>
</goals>
</execution>
Running the automated tests
First we need to build Riftsaw itself, so from the RIFTSAW_SRC directory we run:
mvn clean install
Now in the final step we are going to execute the automated test by running this command from the "integration-tests" directory:
mvn -Dorg.jboss.as.home={path_to_jboss_as}
-Dbpelunit.home={path_to_bpelunit}
-Dorg.jboss.esb.home={path_to_jboss_esb}
-Dorg.jboss.as.config=default
-Ddatabase=hsql -Dws.stack=default -Dreplace.qa.jdbc=false clean install
In the log file you can find the report of the test that was made. It should look similar to this:
deploy:
[echo] Deploy Quickstart_bpel_hello_world
[copy] Copying 1 file to /opt/local/jboss/jboss-5.1.0.GA/server/default/deploy
[echo] Waiting 5 seconds to ensure proper deployment
[echo] Running BPEL Unit test for Quickstart_bpel_hello_world
create.reportsDir:
[mkdir] Created dir: /opt/local/jboss/riftsaw-2.0-CR1-src/integration-tests/target/bpelunit-reports
MEASURECOVERAGE=FALSE
ROOTPATH=/ws/
Test Run Completed. 1 run (0 failures, 0 errors)
undeploy:
[echo] Undeploy Quickstart_bpel_hello_world
[delete] Deleting: /opt/local/jboss/jboss-5.1.0.GA/server/default/deploy/Quickstart_bpel_hello_world-1.jar
[echo] Waiting 5 seconds to ensure proper undeployment
During the test the reports (in TXT and XML format) will be generated in the target/bpelunit-reports directory. The XML report contains extensive information that describe what message were sent to the BPEL engine, what was the expected and received outcome and how it affected the result of the test.
In the log you can see the build waits 5 seconds before and after the test run. This is to ensure the proper deployment. Of course in case there are more tests the deployment wouldn't be made for each single test separately, but for efficiency reasons a deployment of all processes would happen at one moment. In the presented example we use the approach just to make it as simple as possible.
Conclusion
We've just managed to successfully test the Quickstart Hello World process using a simple request-response test via BPELUnit. Transforming other samples to use this tool will be now even more easy, because now after we create the test suite (for example using the Eclipse wizard), we only need to copy the build script file with one small modification – changing the project name. The second piece of code also stays the same and as for the "pom.xml", we just need to add one line for each test.